반응형
strjoin
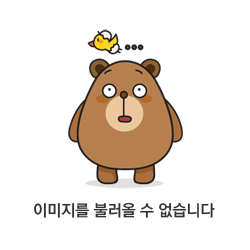
이번에 포인터로 문제를 풀어보았음
이 함수에서는 s1문자열과 s2 문자열을 합해서 리턴을 해야함.
malloc을 사용해 메모리 할당을 받은 공간에 문자를 합쳐서 리턴을 해주어야함.
#include "libft.h"
char *ft_strjoin(char const *s1, char const *s2)
{
int count;
char *temp;
count = strlen(s1);
count += strlen(s2);
temp = (char *)malloc(sizeof(char) * count + 1);
if (!temp)
return (0);
while (*s1)
{
*temp = *s1;
s1++;
temp++;
}
while (*s2)
{
*temp = *s2;
s2++;
temp++;
}
*temp = '\\0';
return ((temp - count));
}
int main()
{
char s[10] = "abc";
char a[10] = "abc";
char *temp;
temp = ft_strjoin(s, a);
printf("aa : %s\\n", temp);
}
itoa
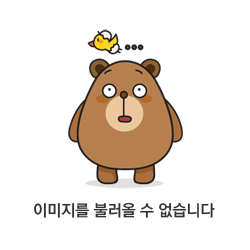
함수 원형
char *ft_itoa(int n)
int형 → 문자열 바꾸기
int형 최대, 최소값 생각해서 캐스팅 해주어야함
#include "libft.h"
long long len(long long count)
{
int num;
num = 0;
if (count < 0)
{
num++;
count *= -1;
}
else if (count == 0)
{
num++;
return (num);
}
while (count > 0)
{
count = count / 10;
num++;
}
return (num);
}
char *ft_c(char *temp)
{
temp[0] = '0';
return (temp);
}
char *ft_itoa(int n)
{
long long count;
int malloc_count;
char *temp;
count = (long long)n;
malloc_count = len(count);
temp = (char *)malloc(sizeof(char) * malloc_count + 1);
if (!temp)
return (0);
temp[malloc_count--] = '\\0';
if (count == 0)
return (ft_c(temp));
if (count < 0)
{
temp[0] = '-';
count *= -1;
}
while (count > 0)
{
temp[malloc_count] = (count % 10) + '0';
count = count / 10;
malloc_count--;
}
return (temp);
}
strmapi
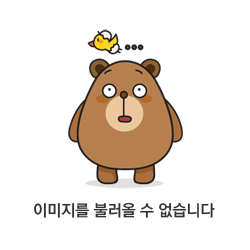
함수 원형
char *ft_strmapi(char const *s, char (*f)(unsigned int, char))
f가 정확하게 무슨 함수인지는 모르나 매개 변수로 unsigned int와 char가 들어가서 리턴값이 char가 나오는 것임
문제에서 f함수에서 반환하는 char를 temp에 넣어서 리턴해야함. 단, malloc사용
#include "libft.h"
char *ft_strmapi(char const *s, char (*f)(unsigned int, char))
{
int i;
char *temp;
int count;
i = 0;
count = strlen(s);
temp = (char *)malloc(sizeof(char) * count + 1);
if (!temp)
return (0);
while (s[i] != '\\0')
{
temp[i] = (*f)(i, s[i]);
i++;
}
temp[i] = '\\0';
return (temp);
}
반응형
'42Seoul > libft' 카테고리의 다른 글
libft - ft_putchar_fd, ft_putstr_fd, ft_putendl_fd, ft_putnbr_fd (0) | 2023.03.24 |
---|---|
libft - strtrim, striteri, split (0) | 2023.03.24 |
libft - strdup, strlen, substr (0) | 2023.03.24 |
libft - strnstr, atoi, calloc (0) | 2023.03.24 |
libft - strrchr, memchr, memcmp (2) | 2023.03.24 |