반응형
strtrim
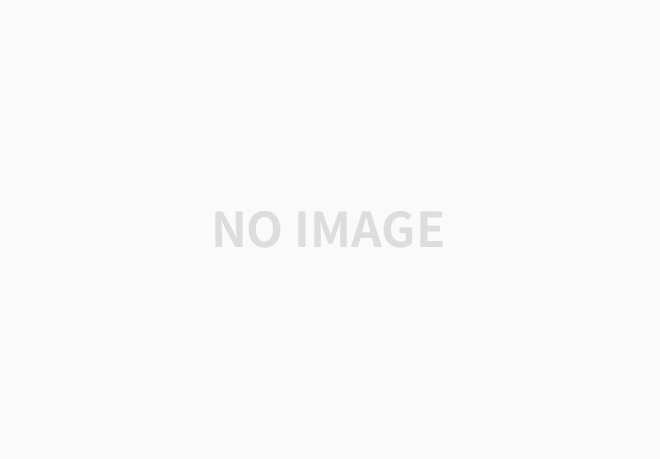
(공백)Hello world! 가 있으면
set : H(공백)e!
첫번 째 시작 점 찾기: (첫 번째) l
끝났으니 뒤에서 부터 마지막 지점 찾기: d
llo world까지 출력
char s[100] = "lorem ipsum dolor sit amet";
char set[6] = "l ";
결과값
:orem ipsum dolor sit amet
char s[100] = " lorem ipsum dolor sit amet ";
char set[6] = "l ";
결과값
:orem ipsum dolor sit amet
실패 코드
#include "libft.h"
int first_word(char const *s1, char const *set)
{
int i;
int j;
j = 0;
i = 0;
while (s1[i] != '\\0')
{
j = 0;
while (set[j] != '\\0')
{
if (s1[i] == set[j])
{
break ;
}
j++;
}
if (s1[i] != set[j])
return (i);
i++;
}
return (0);
}
int second_word(char const *s1, char const *set)
{
int i;
int j;
j = 0;
i = strlen(s1) - 1;
while (s1[i] != '\\0')
{
j = 0;
while (set[j] != '\\0')
{
if (s1[i] == set[j])
{
break ;
}
j++;
}
if (s1[i] != set[j])
return (i);
i--;
}
return (0);
}
int change(int sum)
{
if (sum < 0)
{
return (-sum);
}
return (sum);
}
char *ft_strtrim(char const *s1, char const *set)
{
int first;
int second;
int sum;
char *temp;
int i;
i = 0;
first = first_word(s1, set);
second = second_word(s1, set);
sum = change(first - second);
temp = (char *)malloc(sizeof(char) * sum + 1);
if (!temp)
return (NULL);
if (!set)
return (ft_strdup(s1));
while (sum + 1)
{
temp[i] = s1[first];
i++;
first++;
sum--;
}
temp[i] = '\\0';
return (temp);
}
int main()
{
char s[100] = "lorem ipsum dolor sit amet";
char set[6] = "l ";
char *temp;
temp = ft_strtrim(s, set);
printf("%s", temp);
free(temp);
}
성공 코드
#include "libft.h"
char *ft_strdup(const char *string);
size_t ft_strlen(const char *src);
char *ft_substr(char const *s, unsigned int start, size_t len);
char *ft_strchr(const char *s, int c);
char *ft_strtrim(char const *s1, char const *set)
{
size_t start;
size_t fin;
char *temp;
start = 0;
if (s1 == NULL || set == NULL)
return (0);
fin = ft_strlen(s1);
while (s1[start] && ft_strchr(set, s1[start]))
start++;
while (fin && s1[fin - 1] && ft_strchr(set, s1[fin - 1]))
fin--;
if (start > fin)
return (ft_strdup(""));
temp = ft_substr(s1, start, fin - start);
return (temp);
}
마지막 if 예외 처리는 무엇인가?
start의 길이가 fin보다 길어버리면 스트링 문자열에서 널값을 지난 값을 지칭하고 있다.
그래서 빈 문자열을 처리해주어야한다.
striteri
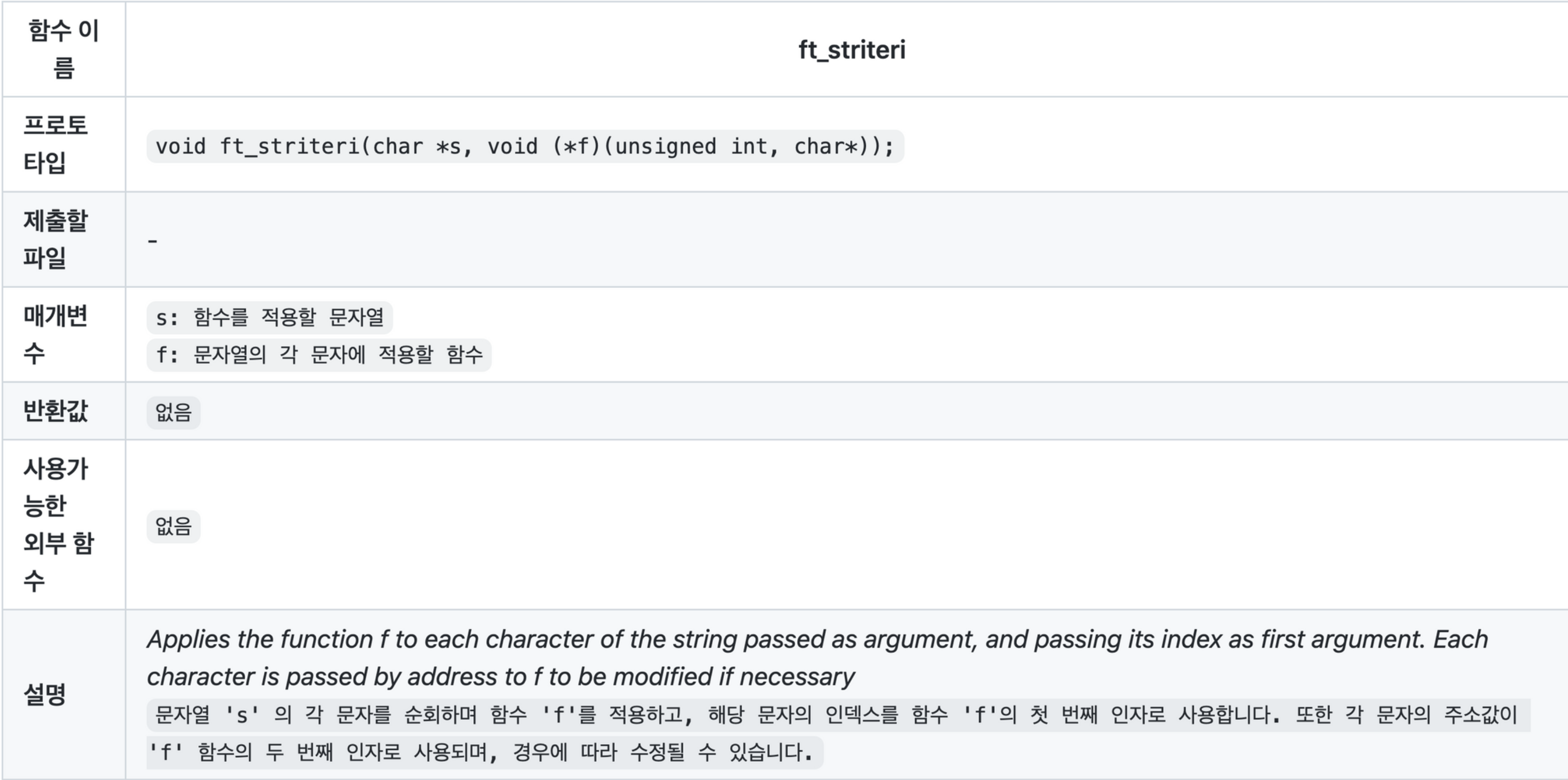
문자열 s가 들어옴. s문자열 길이만큼 f 함수 적용
#include "libft.h"
void ft_striteri(char *s, void (*f)(unsigned int, char *))
{
int i;
i = 0;
while (s[i] != '\\0')
{
(*f)(i, (s + i));
i++;
}
}
split
- 우리가 알던 split과 다름
- 만약 할당을 제대로 못 했을 시 free를 꼭 해주어야함
char s1[20] = "aa aa aa";
char s = ' ';
결과값
2차원 배열에 담긴 aa aa aa가 나와야함.
#include "libft.h"
int string_check(char s, char c)
{
if (s == c)
return (1);
return (0);
}
int malloc_count(char const *s, char c)
{
int i;
int count;
count = 0;
i = 0;
while (s[i] != '\\0')
{
while (s[i] && (string_check(s[i], c) == 1))
i++;
if (string_check(s[i], c) == 0)
{
count++;
i++;
}
while (s[i] && (string_check(s[i], c) == 0))
i++;
}
return (count);
}
char *word_input(char const *s, char c)
{
int i;
char *temp;
int count;
int j;
j = 0;
count = 0;
i = 0;
while (s[i] != '\\0' && string_check(s[i], c) == 0)
{
i++;
count++;
}
temp = (char *)malloc(sizeof(char) * count + 1);
while (s[j] != '\\0' && string_check(s[j], c) == 0)
{
temp[j] = s[j];
j++;
}
temp[j] = '\\0';
return (temp);
}
char **ft_split(char const *s, char c)
{
char **temp;
int count;
int size;
count = 0;
size = malloc_count(s, c);
temp = (char **)malloc(sizeof(char *) * size + 1);
while (*s)
{
while (*s && string_check(*s, c) == 1)
s++;
if (string_check(*s, c) == 0)
{
temp[count] = word_input(s, c);
count++;
s++;
}
while (*s && (string_check(*s, c) == 0))
s++;
}
temp[count] = 0;
return (temp);
}
// "aa aa aa" " "
int main()
{
char s1[20] = "aa aa aa";
char s = ' ';
char **temp = ft_split(s1, s);
int i = 0;
while (temp[i])
{
printf("%s\\n", temp[i]);
i++;
}
free(temp);
}
반응형
'42Seoul > libft' 카테고리의 다른 글
libft Bonus - Makefile (0) | 2023.03.24 |
---|---|
libft - ft_putchar_fd, ft_putstr_fd, ft_putendl_fd, ft_putnbr_fd (0) | 2023.03.24 |
libft - strjoin, itoa, strmapi (0) | 2023.03.24 |
libft - strdup, strlen, substr (0) | 2023.03.24 |
libft - strnstr, atoi, calloc (0) | 2023.03.24 |